NordVPN with Tailscale Exit-Node
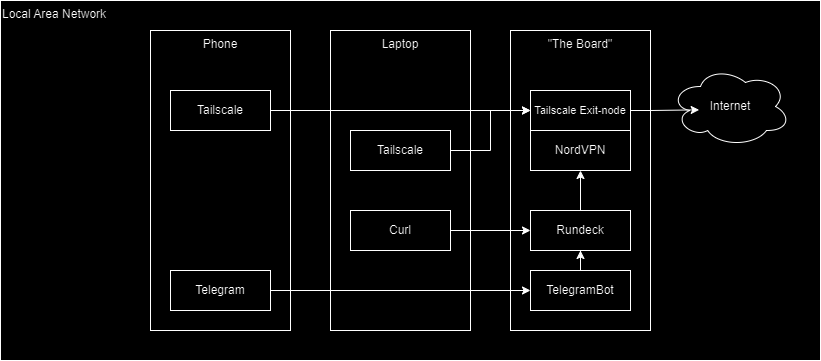
Context
This is for research purposes. I've been utilizing Tailscale's exit node from this blog's virtual machines, which sufficiently hides my internet tracks and improves my internet speed. For some websites, media loads noticeably slower without a VPN. I don't use it to unblock government-restricted sites, as I don't visit those sites anyway ;)
The idea arose because I wondered if it was possible to enhance my online privacy further, as the IP detected on the server side is still associated with this blog, which has my name attached to it.
The big question is: can I achieve more with less cost?
Enter NordVPN + Tailscale
I can set up a virtual machine (VM) without my name attached. A new VM with a public IP will cost me $5 a month from a reliable cloud provider. NordVPN costs $3.69 a month for up to 10 devices. If I find a group of 10 to share a NordVPN subscription, it could cost as low as $0.369 a month. However, seakun.id offers a similar service with a small fee, and I want to set this up quickly, so I'll use seakun.
I'm still limited to one active VPN at a time and I have many devices. I need to set up a "gateway" for all my devices. I already do this with Tailscale's exit node from my cloud VPN, so I just need to set up NordVPN on my Orange Pi (referred to as "the board" from now on) and then use that node as my Tailscale exit node.
# setup nord
sh <(wget -qO - https://downloads.nordcdn.com/apps/linux/install.sh)
nordvpn login
nordvpn login --callback "nordvpn://login?action=login&exchange_token=copythisfromcontinuebuttonrightclickcopylink&status=done"
# ssh routing (optional)
sudo ip route add 192.168.6.0/24 dev eth0 # lan
sudo ip route add 100.64.0.0/10 dev tailscale0 # tailscale
# whitelist so we can ssh
nordvpn whitelist add subnet 100.64.0.0/10 # tailscale
nordvpn whitelist add subnet 192.168.6.0/24 # lan
# tailscale up
echo 'net.ipv4.ip_forward = 1' | sudo tee -a /etc/sysctl.d/99-tailscale.conf\necho 'net.ipv6.conf.all.forwarding = 1' | sudo tee -a /etc/sysctl.d/99-tailscale.conf\nsudo sysctl -p /etc/sysctl.d/99-tailscale.conf
sudo tailscale up --advertise-exit-node
# approve exit node on tailscale console
# disable nordvpn firewall (or allow tailscale network) or tailscale will return offline status with error log: Tailscale hasn't received a network map from the coordination server in
nordvpn set firewall off
# I need to have my tailscale magic dns works, also adguard dns
nordvpn set dns 94.140.14.14 100.100.100.100
nordvpn connect antartica
I can use "the board" as an exit node, and it detects me wherever I connect the NordVPN. However, I encountered a small issue where I couldn't SSH into "the board," which I resolved by downgrading from the latest version.
Enter Rundeck
It annoyed me to SSH into "the board" every time I needed to change the country. To streamline this, I overengineered a solution and set up Rundeck to provide a UI for running NordVPN commands. Now, it looks like this:
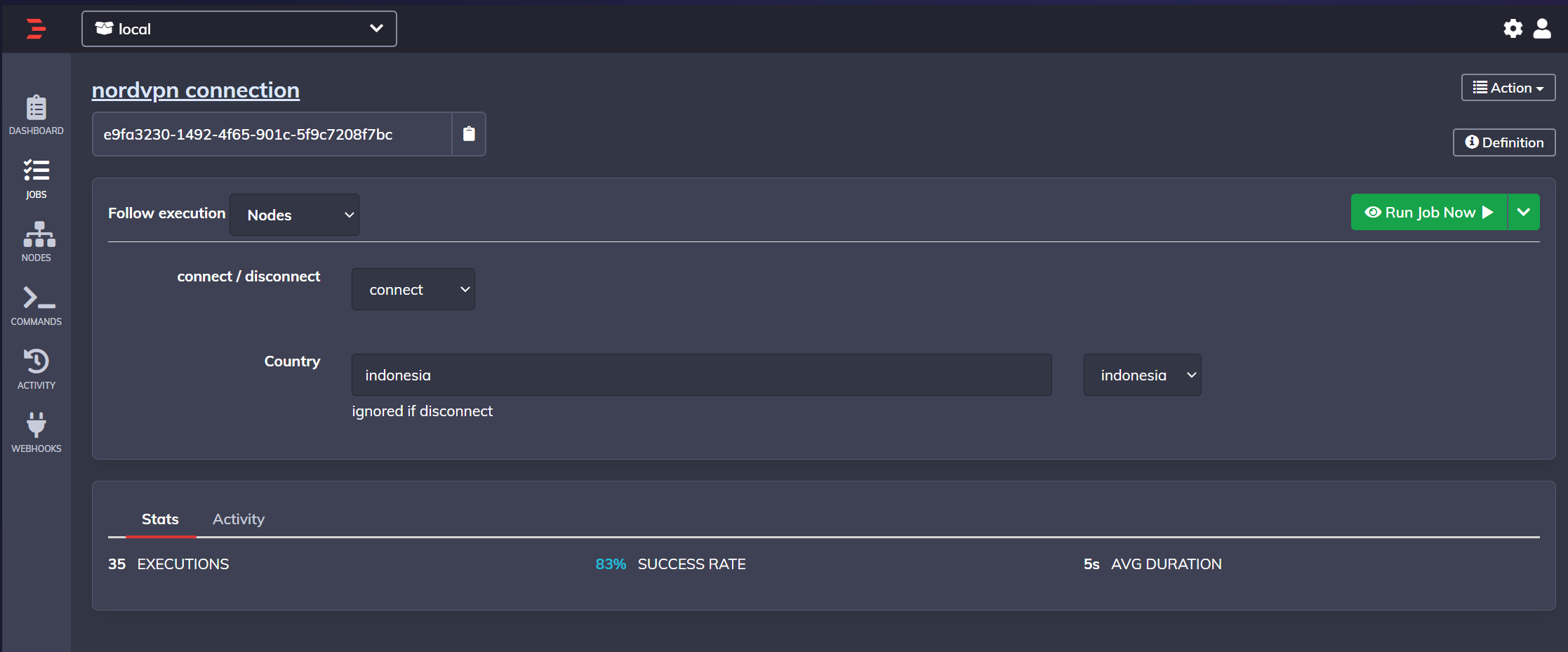
But after a few uses, I found Rundeck wasn't as easy as it seemed. I had to log in and click several things before doing anything. There should be an easier way, right?
Enter Rundeck's API endpoint, With Rundeck's API endpoint, I can run a job with its parameters from a curl command. I just need to generate a token, and I can do this from my terminal. However, a phone doesn't have a terminal. Yes, I know it has Termux, but I've tried it, and it requires more setup to be usable—it doesn't even have history by default. :facepalm:
.. However, a phone doesn't have a terminal. Yes I know it has Termux, but I've tried it, and it requires more setup to be usable—it doesn't even have history by default. :facepalm:
Enter Telegram bot
To complicate this small project, I needed something that could interact with my Rundeck API Gateway or NordVPN directly. Fortunately, among all the free messaging services, Telegram Bot is the easiest to set up, thanks to BotFather. Creating the code wasn't hard, especially with help from ChatGPT. Here's the Python code with some tweaks like I don't want this bot to be accessible to any Telegram user except me:
from telegram import Update, ReplyKeyboardMarkup
from telegram.ext import Application, CommandHandler, MessageHandler, filters, CallbackContext, ConversationHandler
import requests,os
from dotenv import load_dotenv
from functools import wraps
load_dotenv()
# Define states for the conversation
CHOOSING, GET_REGION = range(2)
HEADERS={
"X-Rundeck-Auth-Token": os.getenv('RUNDECK_AUTH_TOKEN'),
"Content-Type": "application/json",
}
# Define the allowed user ID (replace with your actual user ID)
ALLOWED_USER_ID = 123456789 # Replace with your actual Telegram user ID
# Define a decorator to check if the user is allowed
def restricted(func):
@wraps(func)
async def wrapped(update: Update, context: CallbackContext, *args, **kwargs):
if update.effective_user.id != ALLOWED_USER_ID:
await update.message.reply_text("You are not authorized to use this bot.")
return ConversationHandler.END
return await func(update, context, *args, **kwargs)
return wrapped
# Define command handler for /connectvpn
@restricted
async def connectvpn(update: Update, context: CallbackContext) -> int:
reply_keyboard = [['Connect', 'Disconnect']]
await update.message.reply_text(
'Please choose an option:',
reply_markup=ReplyKeyboardMarkup(reply_keyboard, one_time_keyboard=True)
)
return CHOOSING
# Define message handler for the choice
@restricted
async def choice(update: Update, context: CallbackContext) -> int:
user_choice = update.message.text
if user_choice == 'Connect':
await update.message.reply_text('Please enter the region:')
return GET_REGION
elif user_choice == 'Disconnect':
# Perform HTTP request for disconnect
data = {"argString": "-connection disconnect"}
response = requests.post(os.getenv('RUNDECK_VPN_JOB_URL'),headers=HEADERS, json=data)
if response.status_code == 200:
await update.message.reply_text('VPN disconnected successfully.')
else:
await update.message.reply_text('Failed to disconnect VPN.')
return ConversationHandler.END
# Define message handler for the region
@restricted
async def get_region(update: Update, context: CallbackContext) -> int:
region = update.message.text
# Perform HTTP request for connect
data = {"argString": "-connection connect -country " + region}
response = requests.post(os.getenv('RUNDECK_VPN_JOB_URL'),headers=HEADERS, json=data)
if response.status_code == 200:
await update.message.reply_text(f'VPN connected to {region} successfully.')
else:
await update.message.reply_text(f'Failed to connect VPN to {region}.')
return ConversationHandler.END
# Define command handler for /start
async def start(update: Update, context: CallbackContext) -> None:
await update.message.reply_text('Hello! Use /connectvpn to manage your VPN.')
# Define function to cancel the conversation
async def cancel(update: Update, context: CallbackContext) -> int:
await update.message.reply_text('Operation cancelled.')
return ConversationHandler.END
def main():
# Create the Application and pass it your bot's token
# Create the Application and pass it your bot's token
application = Application.builder().token(os.getenv('TELEGRAM_TOKEN')).build()
# Define conversation handler with the states CHOOSING and GET_REGION
conv_handler = ConversationHandler(
entry_points=[CommandHandler('connectvpn', connectvpn)],
states={
CHOOSING: [MessageHandler(filters.Regex('^(Connect|Disconnect)$'), choice)],
GET_REGION: [MessageHandler(filters.TEXT & ~filters.COMMAND, get_region)]
},
fallbacks=[CommandHandler('cancel', cancel)]
)
# Register handlers
application.add_handler(conv_handler)
application.add_handler(CommandHandler("start", start))
# Start the Bot
application.run_polling()
if __name__ == '__main__':
main()